Verifying QRs
The official GreenPass apps are usually of two kinds:
- wallet-like (can store certificates)
- verifier-like (can NOT persist certificates)
I only guess that this decision was made to ban official forces who use verifier apps to store personal data of verified people. On the other side, there is no such protection for this in wallet apps (so everybody can scan and save QR code of his / her friend) it's still the responsibility of the authorities to ask for ID card, etc.
#
Verify ExampleBluePass-19 verifies the scanned QRs in accordance with official (country level) apps (for further specification, see motivation page).
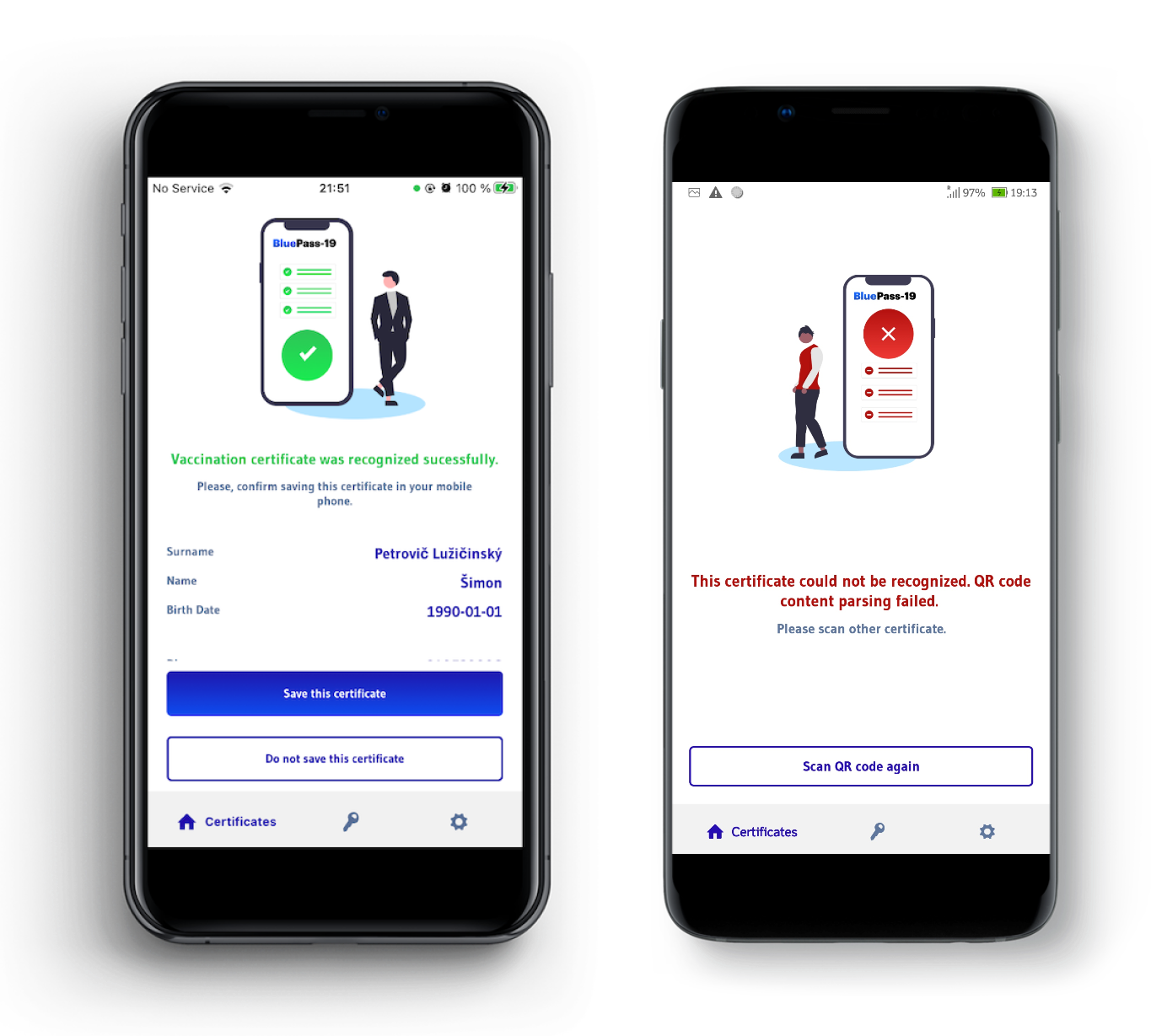
#
Verify FlowHere is an explanation of how QR codes are verified. Any thrown errors are mapped to bussiness / UI errors in accordance to this object on next page.
#
Verify Pseudocode- Strip HC:1 prefix : "HC:1" prefix is removed from scanned from scanned data.
- Decode by using base45
- Decompress by Zlib
- Decompress by CBOR - returns COSE header, body and signature
- Take KID (Public Key Identifier) from header
- Decode COSE body using CBOR (second time) - return GreenCertificate / Greenpass JSON
- Validate GreenPass by JSON Schema
- Validate IssueDate and ExpiryDate in GreenCertificate header
- Validate signatures using KID from previous steps (fetch public key by KID, and do crypto verification)
- Validate GreenPass by Business rules in JsonLogic
- Validation finished without error - GreenPass is valid
#
JS CodeIf you prefer code, take a look below:
If you are interested in libraries (dependencies), that were used in React Native, see Installation section.
#
Browsing Stored CertificatesIf user scanned valid QR certificate, he will have the opportunity to persist it for later use. All stored certificates could be found at the home screen.
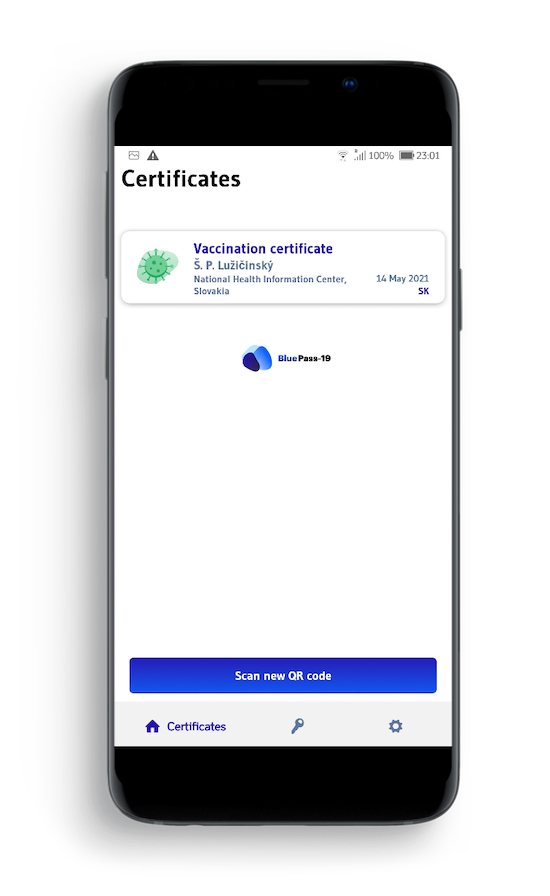
#
Opening Scanned & Stored CertificateIf authorities would require user to present his certificate, user can open stored certificate where he will see original QR code with all details that are embedded in that certificate.
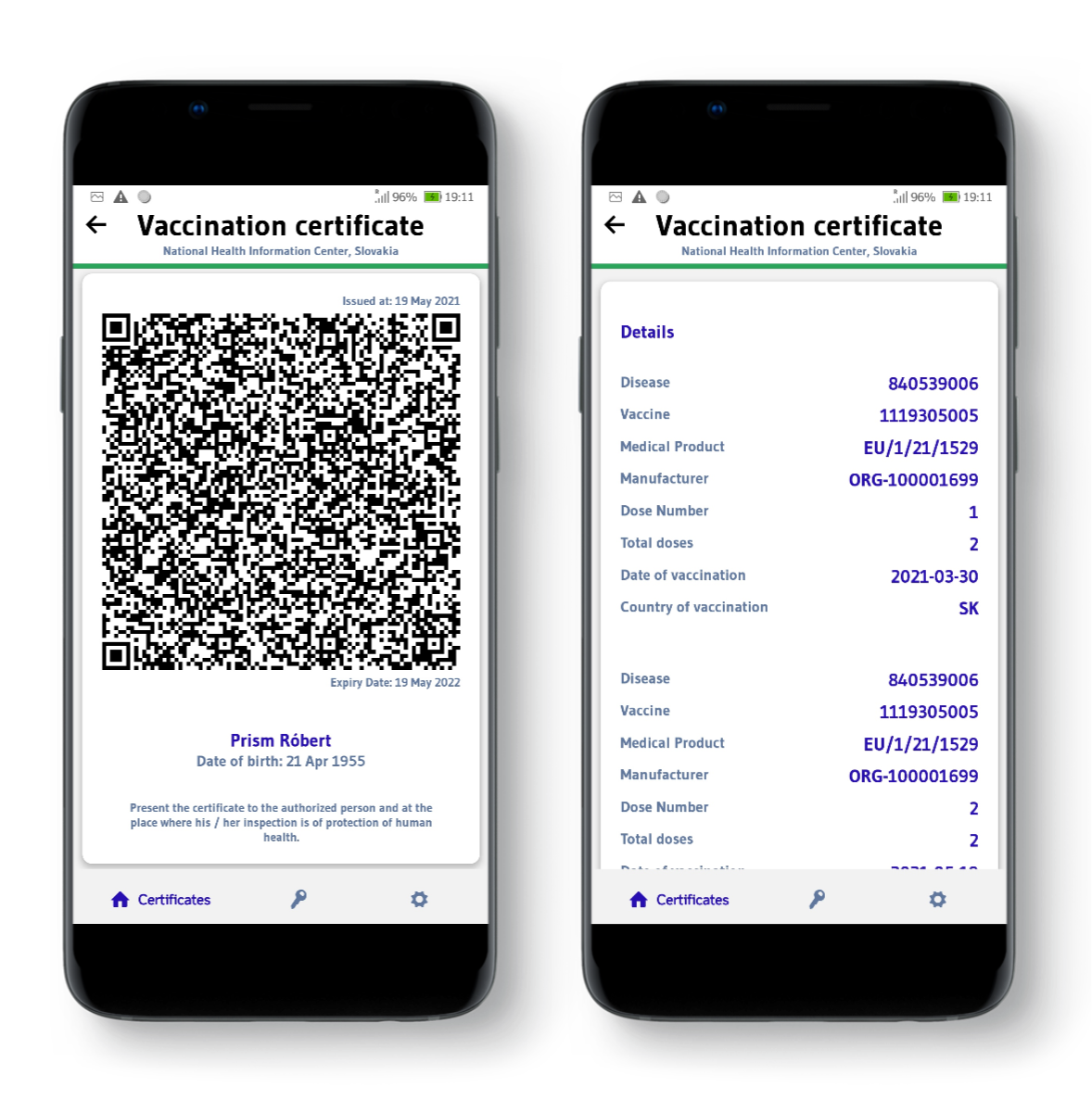